LLUserReactionCounts
is rendered as a message item footer and represents the reaction picker, reactions and reaction count details
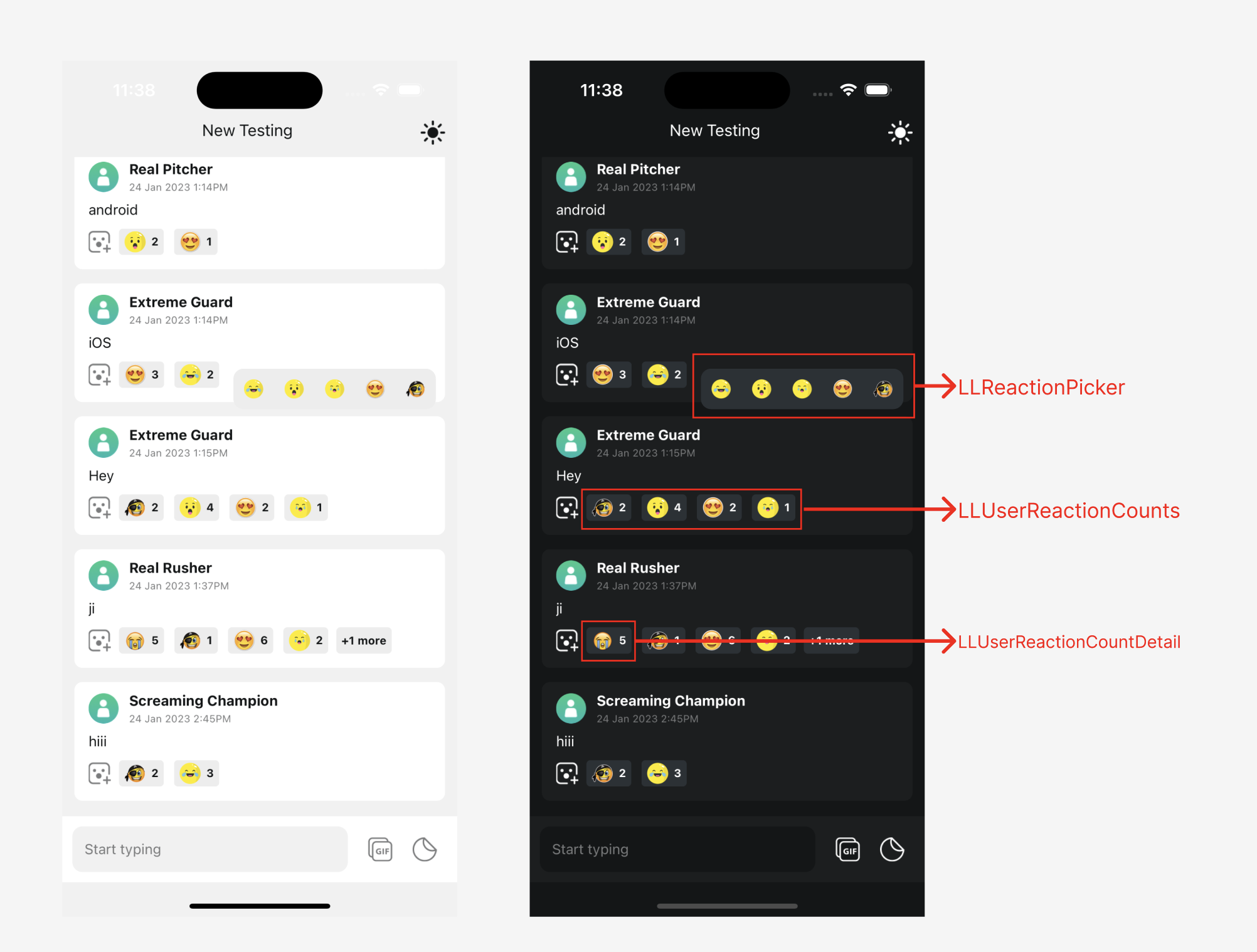
import React from 'react';
import {
LLChat,
LLChatMessageItem,
LLChatMessageItemFooter,
LLChatMessageItemFooterProps,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
LLReactionPickerProps,
LLUserReactionCountDetailProps,
LLUserReactionCounts,
LLUserReactionCountsProps,
} from '@livelike/react-native';
function MyUserReactionsPickerComponent(props: LLReactionPickerProps) {
// render custom reaction picker component
}
function MyUserReactionsCountDetailComponent(
props: LLUserReactionCountDetailProps
) {
// render custom reaction count detail component
}
function MyUserReactionsCountComponent(props: LLUserReactionCountsProps) {
return (
<LLUserReactionCounts
{...props}
MessageReactionPickerComponent={MyUserReactionsPickerComponent}
UserReactionCountDetailComponent={MyUserReactionsCountDetailComponent}
/>
);
}
function MyMessageItemFooter(props: LLChatMessageItemFooterProps) {
return (
<LLChatMessageItemFooter
{...props}
UserReactionsCountComponent={MyUserReactionsCountComponent}
/>
);
}
function MyMessageItemComponent(props: LLChatMessageItemProps) {
return (
<LLChatMessageItem
{...props}
MessageItemFooterComponent={MyMessageItemFooter}
/>
);
}
function MyMessageListComponent(props: LLChatMessageListProps) {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
}
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
import React from 'react';
import {
LLChat,
LLChatMessageItem,
LLChatMessageItemFooter,
LLChatMessageItemFooterProps,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
LLReactionPicker,
LLReactionPickerItemStyles,
LLReactionPickerProps,
LLReactionPickerStyles,
LLUserReactionCountDetailStyles,
LLUserReactionCounts,
LLUserReactionCountsProps,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
function MyUserReactionsPickerComponent(props: LLReactionPickerProps) {
return (
<LLReactionPicker
{...props}
styles={reactionPickerStyle}
ReactionItemComponentStyles={reactionItemStyles}
/>
);
}
function MyUserReactionsCountComponent(props: LLUserReactionCountsProps) {
return (
<LLUserReactionCounts
{...props}
MessageReactionPickerComponent={MyUserReactionsPickerComponent}
UserReactionCountDetailComponentStyles={userReactionCountDetailStyles}
/>
);
}
function MyMessageItemFooter(props: LLChatMessageItemFooterProps) {
return (
<LLChatMessageItemFooter
{...props}
UserReactionsCountComponent={MyUserReactionsCountComponent}
/>
);
}
function MyMessageItemComponent(props: LLChatMessageItemProps) {
return (
<LLChatMessageItem
{...props}
MessageItemFooterComponent={MyMessageItemFooter}
/>
);
}
function MyMessageListComponent(props: LLChatMessageListProps) {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
}
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
const reactionPickerStyle: Partial<LLReactionPickerStyles> = StyleSheet.create({
pickerContainer: { bottom: 0, right: 0, borderRadius: 10 },
});
const reactionItemStyles: Partial<LLReactionPickerItemStyles> =
StyleSheet.create({
reactionIcon: { height: 12, width: 12 },
});
const userReactionCountDetailStyles: Partial<LLUserReactionCountDetailStyles> =
StyleSheet.create({
reactionCountText: { fontSize: 9 },
reactionIcon: { height: 12, width: 12 },
});
Type | Default |
---|
String (Required) | No Default |
Type | Default |
---|
String (Required) | No Default |
Type | Default |
---|
boolean | false if not present |
Type |
---|
Function of type: (reactionId: string) => void (Required) |
Type | Default |
---|
StyleSheet of type LLUserReactionCountsStyles | No Default, if present styles props would be applied on top of internal LLUserReactionCounts styles. |
CSS Class | Type | Description |
---|
reactionCountsContainer | ViewStyle | Reaction counts root container |
moreReactionsView | ViewStyle | More reactions button container |
moreReactionsText | TextStyle | More reactions button text styles |
LLUserReactionCountDetail
represents a single reaction in reaction counts list
Type |
---|
Function of type: (reactionId: string) => void (Required) |
Type | Default |
---|
StyleSheet of type LLUserReactionCountDetailStyles | No Default, if present styles props would be applied on top of internal LLUserReactionCountDetail styles. |
CSS Class | Type | Description |
---|
container | ViewStyle | Root reaction container |
reactionIcon | ImageStyle | Reaction icon styles |
reactionCountText | TextStyle | Reaction count text styles |
selfReactionContainer | ViewStyle | Self reaction root container |
selfReactionCountText | TextStyle | Self reaction count text styles |
LLReactionPicker
renders the reaction picker when user press on add reaction icon in message item footer
Type | Default |
---|
boolean | False if not present |
Type | Default |
---|
String (Required) | No Default |
Type |
---|
Function of type: (reactionId: string) => void (Required) |
Type | Default |
---|
LLReactionPickerItemStyles | No Default, if present styles props would be applied on top of internal LLReactionPickerItem styles. |
Type | Default |
---|
StyleSheet of type LLReactionPickerStyles | No Default, if present styles props would be applied on top of internal LLReactionPicker styles. |
CSS Class | Type | Description |
---|
pickerContainer | ViewStyle | Root reactions picker container |
LLReactionPickerItem
renders a single reaction in reaction picker
Type |
---|
Function of type: (id: string) => void (Required) |
Type | Default |
---|
StyleSheet of type LLReactionPickerItemStyles | No Default, if present styles props would be applied on top of internal LLReactionPickerItem styles. |
CSS Class | Type | Description |
---|
reactionIcon | ImageStyle | Reaction icon styles (in reaction picker) |