Chat Configuration
Update Chat Nickname
When you initialize Chat the user will have a default nickname. If you want to customize it to match your application's username or provide a way to manually set it, you can use the following method.
sdk.profile().updateChatNickname(nickname)
Update User Avatar
You can update the user avatar at the chat room level.
We have a default image for an avatar for placeholder and error. To set your own default image just add an image in drawable named "default_avatar.png" to override the SDK default avatar image
chatsession.avatarUrl="<url>"
chatsession.setAvatarUrl("<url>")
Chat Avatar Toggle
You can toggle the showing of the user avatar at the chat room level.
chatView.shouldDisplayAvatar= <true|false>
chatsession.setShouldDisplayAvatar(<true|false>)
Chat Input Visibility
In a scenario where you wish to create what is called Influencer Chat, you as an integrator have the ability to create a chat experience where a user does not have an option to send any messages only view the incoming ones.
chat_view.isChatInputVisible = true // Hides the chat input
Show Url as HyperLink in ChatView
In a scenario where you wish to show URL as a hyperlink in ChatView.
chatView.enableChatMessageURLs = true
Configure the Sticker Keyboard
To add or remove Stickers from the keyboard, you will need to share sticker packs with LiveLike. See Chat Sticker Guidelines for more details.
Get Unread Message Count Between Sessions
To count unread messages between app sessions you need to keep track of the timestamp of the first unread message received on each channel. With this timestamp you will call the Join API with parameter startTimeStamp to resume the stream of messages from the last timestamp or can use in combination of Count API
- Maintain a dictionary of the timestamps of the first unread message of each room id
- Add to dict when a new message has been received on a channel that is not being displayed
- Remove from dict at roomID when you’ve entered the room at roomID
- Save data to persistent storage to restore after the app closes or goes to background.
chatSession.joinChatRoom("<custom-room-id>", < unix startTimestamp>)
privateGroupChatsession?.setMessageListener(object : MessageListener {
override fun onNewMessage(chatRoom: String, message: LiveLikeChatMessage) {
chatRoomLastTimeStampMap[chatRoom] = Calendar.getInstance().timeInMillis
if (chatRoom == privateGroupChatsession?.getActiveChatRoom?.invoke()) {
messageCount[chatRoom] = mutableSetOf() // reset unread message count
chatRoomLastTimeStampMap[chatRoom] = Calendar.getInstance().timeInMillis
} else {
if (messageCount[chatRoom] == null) {
messageCount[chatRoom] = mutableSetOf(message.id.toString())
} else {
messageCount[chatRoom]?.add(message.id.toString())
}
}
getSharedPreferences("test-app", Context.MODE_PRIVATE).edit().putString("unread_count", GsonBuilder().create().toJson(messageCount)).apply(
}
})
chatSession.joinChatRoom("<custom-room-id>");
chatSession.setMessageListener(
new MessageListener() {
@Override
public void onNewMessage(@NotNull String chatRoom, @NotNull LiveLikeChatMessage message) {
chatRoomLastTimeStampMap[chatRoom] = Calendar.getInstance().timeInMillis
if (chatRoom == chatSession.getActiveChatRoom.invoke()) {
messageCount[chatRoom] = mutableSetOf() // reset unread message count
chatRoomLastTimeStampMap[chatRoom] = Calendar.getInstance().timeInMillis
} else {
if (messageCount[chatRoom] == null) {
messageCount[chatRoom] = mutableSetOf(message.id.toString())
} else {
messageCount[chatRoom] ?.add(message.id.toString())
}
}
getSharedPreferences("test-app", Context.MODE_PRIVATE).edit().putString("unread_count", GsonBuilder().create().toJson(messageCount)).apply(
}
});
Custom chat room inside a session
If the integrator doesn’t want to show the public chat. In order to achieve this, they should set the custom chat room before setting it to the chat_view.
val chatSession = engagementSDK.createContentSession("<chat-program-id>")
chatSession.enterChatRoom("<custom-room-id>")
chatSession.joinChatRoom("<custom-room-id>", @optional startTimestamp)
LiveLikeChatSession chatSession = engagementSDK.createChatSession("<chat-program-id>");
chatSession.enterChatRoom("<custom-room-id>");
chatSession.joinChatRoom("<custom-room-id>", @optional startTimestamp);
startTimestamp is optional-field, it can be used to replay history to handle various use cases like calculating count between session
Chat: Message Timestamp Display
Override Default formatter
class ChatViewWrapper(context: Context, private val attrs: AttributeSet?) : ChatView(context, attrs) {
override fun formatMessageDateTime(messageUnixTimeStamp: Long): String {
return customFormatter.fromat(messageUnixTimeStamp)
}
}
class ChatViewWrapper extends ChatView{
public ChatViewWrapper(@NotNull Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
@NotNull
@Override
public String formatMessageDateTime(@Nullable Long messageTimeStamp) {
return customFormatter.formatMessageDateTime(messageTimeStamp);
}
}
External Keyboard Support
Users can add image/emoji/gif coming from from any custom keyboard (Bijmoji, Giphy, Google Keyboard, etc. )
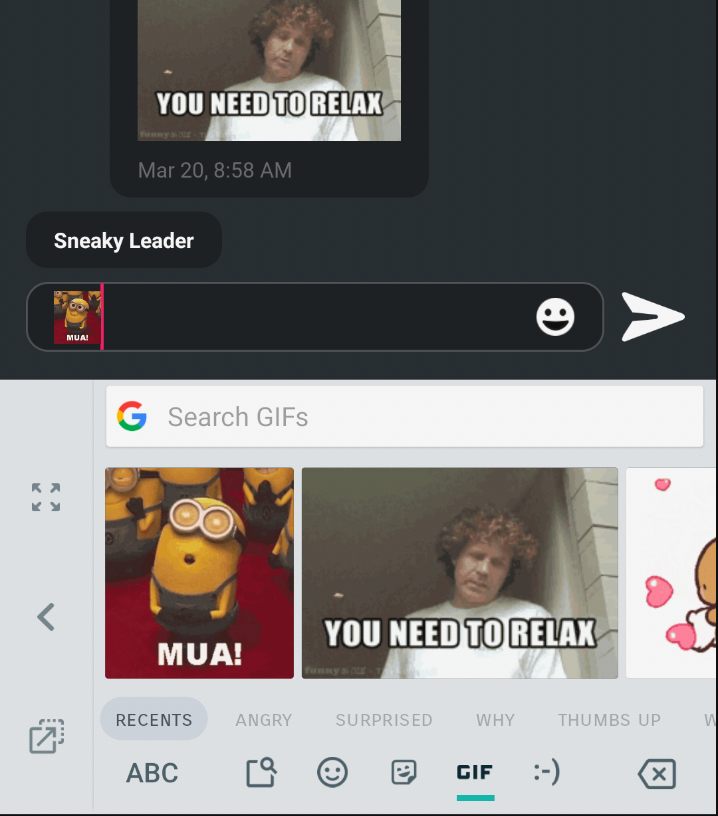
Gboard in action
As an integrator you have the control about activating or deactivating this feature
As soon as your chat view is instantiated you can call the following
// Enable the External Keyboard Support
chat_view.allowMediaFromKeyboard = true
// Disable the External Keyboard Support
chat_view.allowMediaFromKeyboard = false
As soon as your chat view is instantiated you can call the following
// Enable the External Keyboard Support
chat_view.allowMediaFromKeyboard = true;
// Disable the External Keyboard Support
chat_view.allowMediaFromKeyboard = false;
Hide Reaction Panel
To hide the reaction panel in the chat view,integrator can call the below method
chat_view.hidePopUpReactionPanel()
Updated about 1 year ago