LLStickerPicker
LLGifPicker
renders a Sticker picker component when a user press on sticker-picker icon in composer
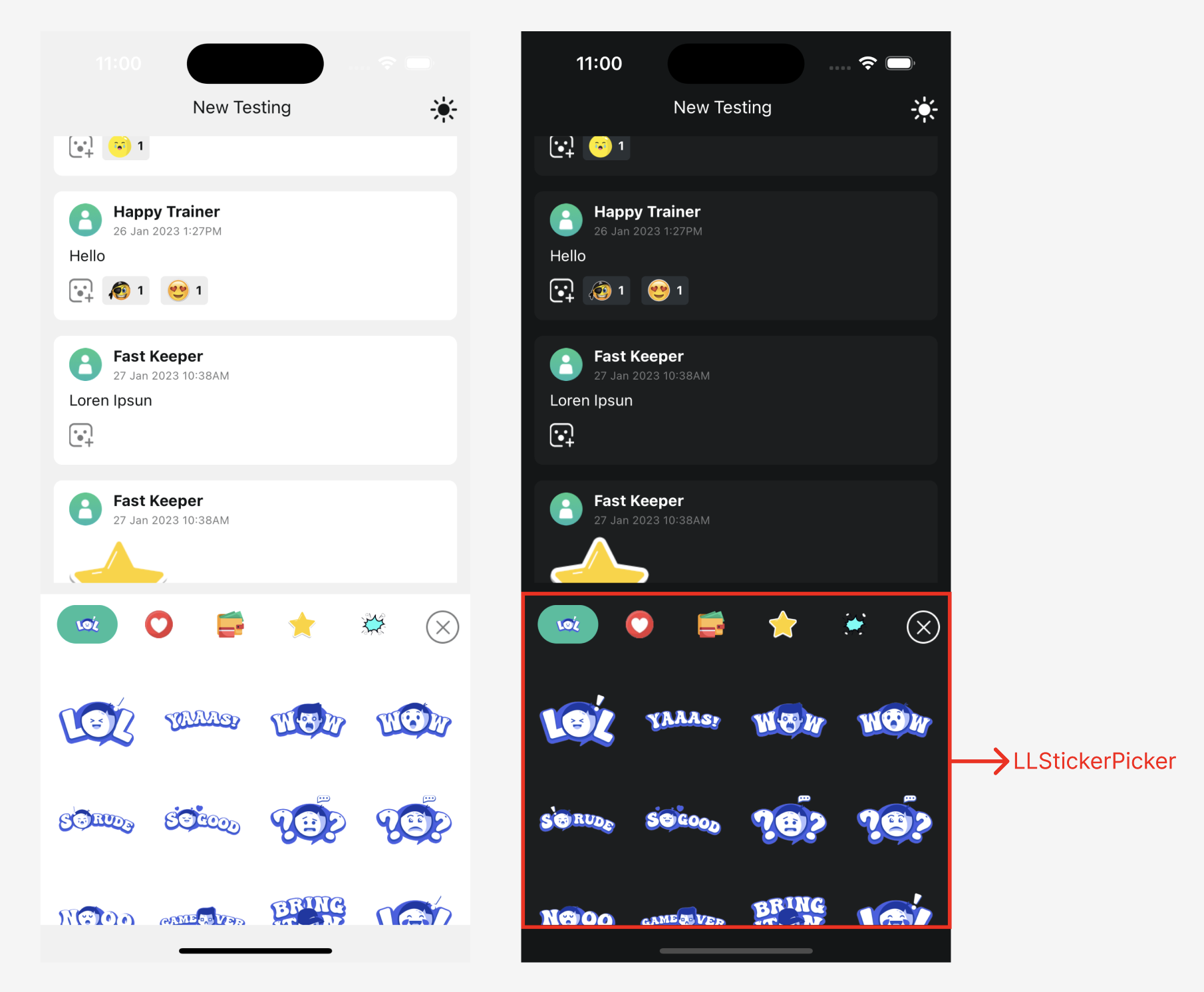
Customise styles for Stock LLStickerPicker
component example:
LLStickerPicker
component example:import React from 'react';
import {
LLBasePickerStyles,
LLChat,
LLChatMessageComposer,
LLChatMessageComposerProps,
LLStickerPicker,
LLStickerPickerProps,
LLStickerPickerStyles,
useTheme,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
import { useMemo } from 'react';
function MyStickerPicker(props: LLStickerPickerProps) {
const { themeType } = useTheme();
const pickerComponentStyles = useMemo(() => pickerComponentStylesFn(themeType), [themeType]);
return (
<LLStickerPicker
{...props}
PickerComponentStyles={pickerComponentStyles}
styles={gifPickerStyles}
/>
);
}
function MyComposer(props: LLChatMessageComposerProps) {
return (
<LLChatMessageComposer
{...props}
StickerPickerComponent={MyStickerPicker}
/>
);
}
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageComposerComponent={MyComposer}
/>
);
}
const gifPickerStyles: Partial<LLStickerPickerStyles> = StyleSheet.create({
pickerCloseIcon: { height: 12, width: 12 },
stickerImage: { width: 70, height: 70 },
stickerPackIcon: { height: 22, width: 22 },
});
const pickerComponentStylesFn: (
theme: 'light' | 'dark'
) => Partial<LLBasePickerStyles> = (theme) =>
StyleSheet.create({
pickerContainer: {
minHeight: 250,
maxHeight: 350,
backgroundColor: theme === 'light' ? '#A0C3D2' : '#2b4956',
},
pickerItemsScrollview: {
padding: 10,
},
});
Hooks used by LLStickerPicker
LLStickerPicker Props
visible
visible
Type | Default |
---|---|
boolean | false if not present |
closeStickerPicker
closeStickerPicker
Type |
---|
Function of type: () => void |
onSelectSticker
onSelectSticker
Type |
---|
Function of type: (stickerShortcode: string) => void (Required) |
PickerComponentStyles
PickerComponentStyles
Type | Default |
---|---|
LLBasePickerStyles | No Default, if present styles props would be applied on top of internal LLBasePicker styles. |
styles
styles
StyleSheet of type LLStickerPickerStyles | No Default, if present styles props would be applied on top of internal LLStickerPicker styles. |
Styles Props
CSS Class | Type | Description |
---|---|---|
stickerPacksContainer | ViewStyle | Sticker packs item container |
stickerImageContainer | ViewStyle | Sticker item container |
stickerHeaderContainer | ViewStyle | Root sticker packs container |
stickerPackIcon | ImageStyle | Sticker packs item styles |
stickerImage | ImageStyle | Sticker item styles |
pickerCloseIcon | ImageStyle | Sticker picker close icon styles |
Updated 12 months ago
What’s Next