Comments
Comment Feature Functionalities
The <livelike-comments>
element will insert a fully-functional comment board into your web application, empowering users with features like moderation, reactions, and profanity filtering for engaging discussions.
a. Comment Board
-boardId
Attribute
boardId
AttributeTo display the complete Comment Feature UI, add the boardId
attribute to an HTML element, specifying the board's ID. The boardId
attribute is required and must be a valid board ID. Comment boards can be created through the API
<script>
LiveLike.init({ clientId })
</script>
<livelike-comments boardId="COMMENT_BOARD_ID"></livelike-comments>
Reaction space to can be added to comments using create reaction space API
- Displaying Comments
Users can view comments posted by others. The Comment Board displays comments in a structured and user-friendly format.
- Posting Comments
Users can contribute to the discussion by posting comments. They can express their thoughts, ask questions, or share opinions.
- Reacting to Comments
Users can react to comments using emojis or custom reaction icons. This feature allows users to express their emotions or agreement with a comment.
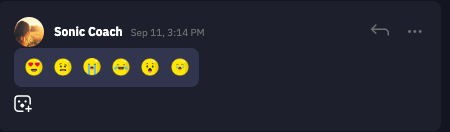
- Replying to Comments
<livelike-comments>
supports threaded discussions, enabling users to reply to specific comments. This fosters more in-depth conversations and keeps discussions organised.
- Sorting Comments
Users can sort comments from newest to oldest or oldest to newest. By default ,the comments will be sorted from newest to oldest.
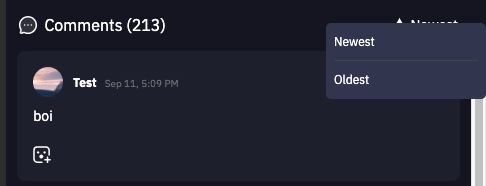
- Pagination
Maximum of 20 comments will be visible on first load. User can load more comments by clicking Load More
button at end of comments list.
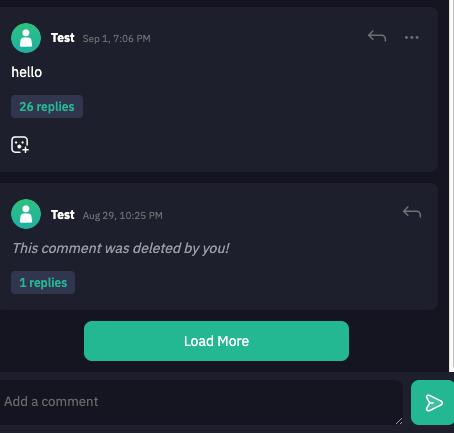
b. Moderation
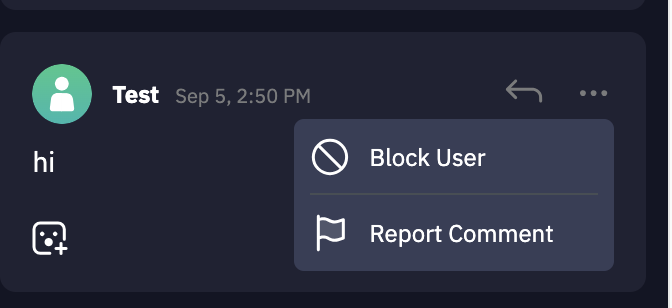
- Reporting Comments
Users can report comments that they find inappropriate or offensive. This feature helps identify and address problematic content.
- Blocking Users
Blocking a user prevents them from replying further on your comment. It's a powerful tool to manage troublesome users.
- Deleting Comments
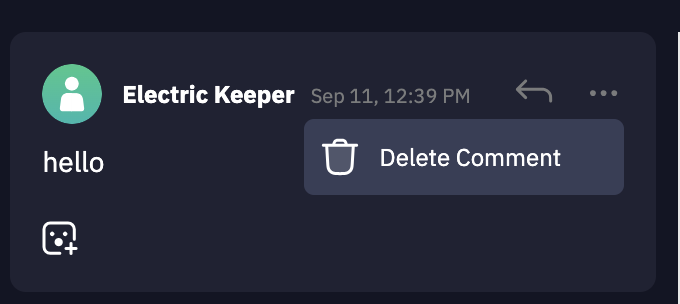
Moderators or authorised users can delete comments when necessary.User can also delete self comment. This ensures that inappropriate or harmful comments can be removed promptly.
c. Profanity Filtering
- Enabling Profanity Filtering
You can enable profanity filtering to automatically detect and hide profane language in comments. To enable profanity filtering, you need to pass contentFilter: 'filtered'
to createCommentBoard or updateCommentBoard API.
LiveLike.createCommentBoard({
title: 'sd',
customId:'customId',
repliesDepth:2,
allowComments:true,
description: 'desc',
customData:'abc',
contentFilter: 'filtered'
}).then(commentBoard => console.log(commentBoard));
When filtering is enabled, comments containing profane/obscene words will be filtered out.
profaneComment
Attribute
profaneComment
AttributeThe profaneComment
attribute allows you to control how profane comments are displayed. You can configure the behaviour of profaneComment either masked with asterisks (e.g., ***
) or according to your custom filter function.
- If you set
profaneComment="mask"
, profane words in the comments will be displayed as asterisks (***
).
<livelike-comments boardId="COMMENT_BOARD_ID" profaneComment="mask"></livelike-comments>
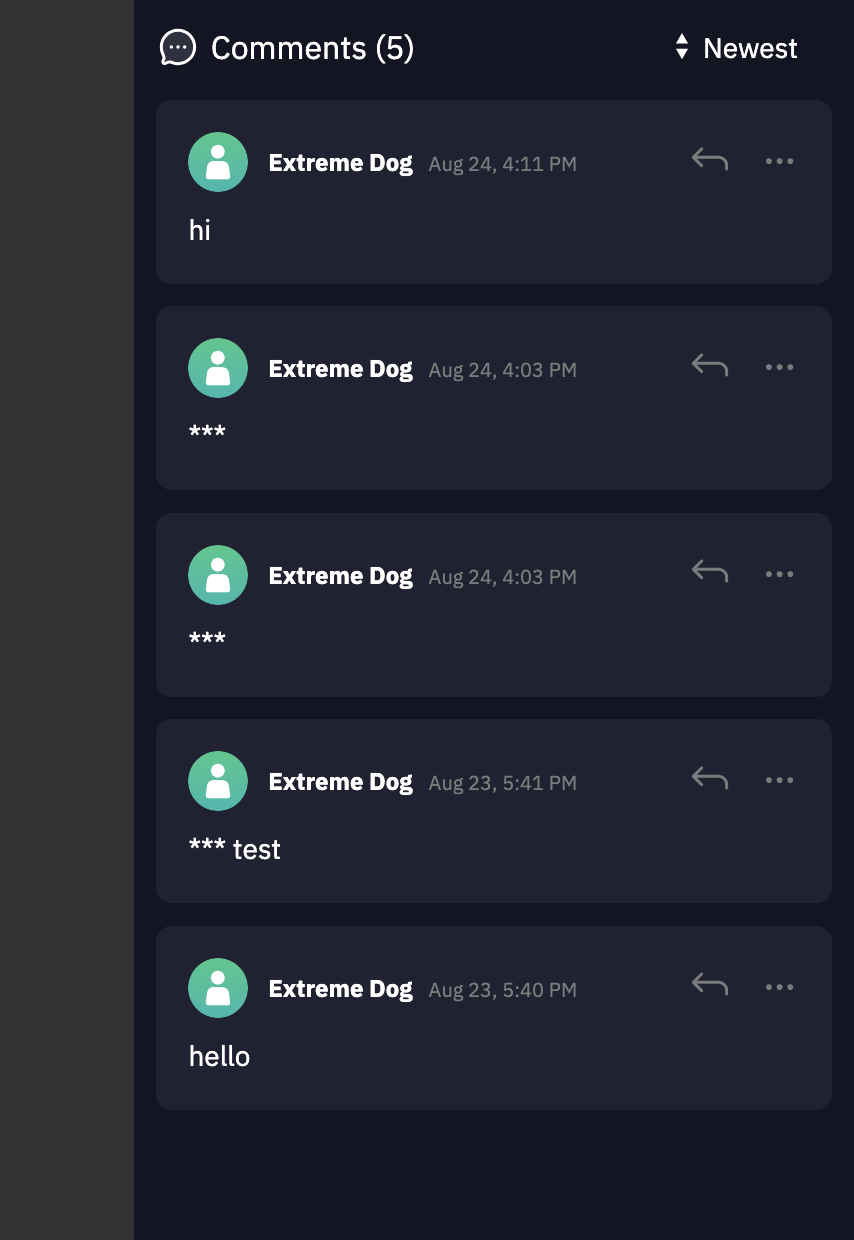
- Custom Profanity Filter Function
If you have specific requirements for profanity filtering, you can define a custom filter function. This function allows you to implement your filtering logic.
- If you set
profaneComment="custom"
, you can provide your custom logic to handle profane comment usingprofaneCommentFilterFunction
attribute.
<livelike-comments boardId="COMMENT_BOARD_ID" profaneComment="custom" profaneCommentFilterFunction={someFunc} >
</livelike-comments>
d. Additional Features
- Update/Add Author Image
Users can associate an image with their comment by providing an authorImageUrl
. This personalizes their comments and adds visual context to their contributions.
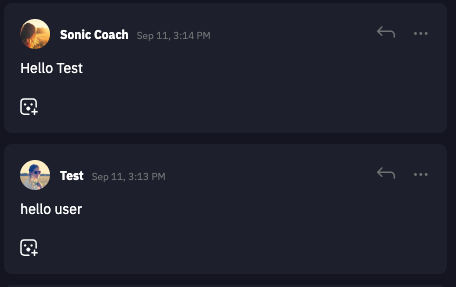
authorImageUrl
Attribute
authorImageUrl
AttributeYou can also specify an author's image URL using the authorImageUrl
attribute.
<livelike-comments boardId="COMMENT_BOARD_ID" authorImageUrl="URL_TO_AUTHOR_IMAGE"></livelike-comments>
authorImageUrl
can also be passed as a param in the comment board url.
- Reaction Packs
<livelike-comments>
support predefined sets of reactions or emojis that users can choose from when reacting to comments. This can add fun and engagement to your comment system. Reaction space to can be added to comments using create reaction space API. Assign the comment board Id to targetGroupId
attribute.
LiveLike.createReactionSpace({
targetGroupId: '603b6329-b682-4af6-86ee-3c10440b6941',
reactionPackIds: ['0affb9e4-94fc-4fe6-b8bd-70f1d67f26cc'],
}).then((reactionSpace) => console.log(reactionSpace));
Creating a reaction space for a comment board is a one time task that you have to do whenever a comment board is been created
Customisation
You can customise the appearance and behaviour of the Comment Board to match your application's branding and user experience. This typically involves modifying CSS styles and using event handling to implement custom logic.
a. Customise CSS
Styling can be updated using css classes and selectors.
livelike-comments{
width: 100%;
}
livelike-comment-board-header{
...
}
Refer stackblitz code for customised css.
b. Customise State
You can customise the behaviour and working of a comment board to suit your requirements. This can be done using controller methods and API methods.
const response = await LiveLike.getComments({ordering:"-created_at",since:"2023-08-22T14:30:45.123Z"})
const filteredComments = LiveLike.commentController.filterComments({
blockedProfileIds: new Set(Livelike._$$.blockedProfileIds),
comments: response.data.results ?? [],
});
const currentState = LiveLike.commentController.getState(commentBoardId);
const newState = { ...currentState, comments: updatedComments };
LiveLike.commentController.setState({
commentBoardId: commentBoardId,
newState: newState
});
In the above example getComments is API method for fetching comments and filterComments is a controller method for filtering blocked profile messages.
You can also update the author image url attribute using controller method updateCommentConfigAction
.
LiveLike.commentController.updateCommentConfig({
commentBoardId: 'c5d2776f-63ea-4bfb-9c3b-ff016a61303b',
commentBoardConfig: {
authorImageUrl: 'https://picsum.photos/id/64/200/200',
},
});
Updated 11 months ago