LLChatHeader
LLChatHeader
represents the ChatUI Header and it is rendered at the top of the Chat UI
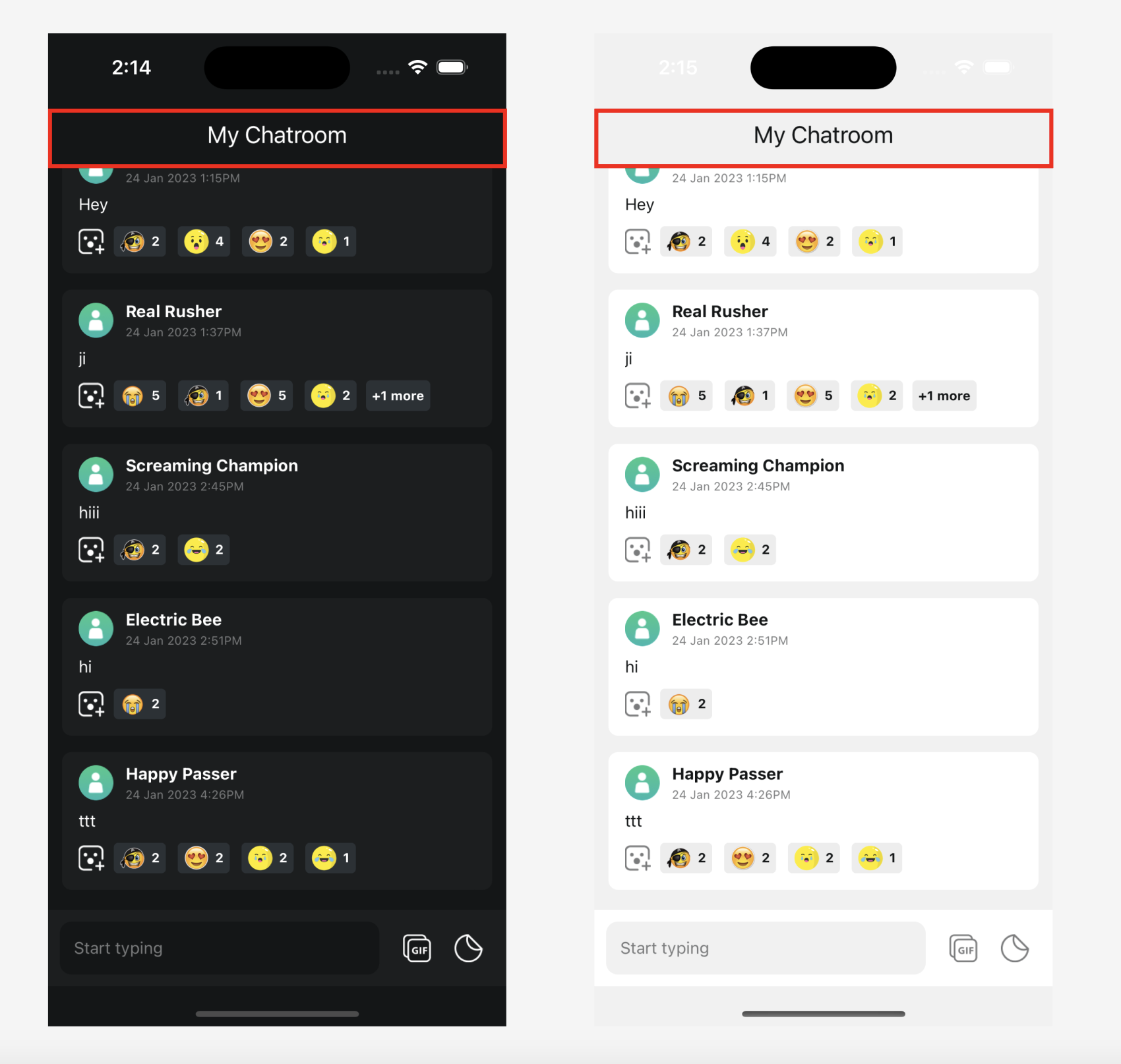
Standalone example usage:
import React from 'react';
import { StyleSheet, View } from 'react-native';
import { LLChatHeader, LLChatHeaderStyles } from '@livelike/react-native';
import { Body, Footer } from '../components';
export function MyApp() {
return (
<View>
<LLChatHeader title="My Header" styles={headerStyle} />
<Body />
<Footer />
</View>
);
}
const headerStyle: Partial<LLChatHeaderStyles> = StyleSheet.create({
headerContainer: { padding: 10 },
headerTitle: { fontSize: 15 },
});
Usage for customising ChatHeader
for ChatUI:
ChatHeader
for ChatUI:import React from 'react';
import {
LLChat,
LLChatHeader,
LLChatHeaderStyles,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
HeaderComponent={() => (
<LLChatHeader title="My Chatroom" styles={headerStyle} />
)}
/>
);
}
const headerStyle: Partial<LLChatHeaderStyles> = StyleSheet.create({
headerContainer: { padding: 10 },
headerTitle: { fontSize: 15 },
});
Hooks used by LLChatHeader
LLChatHeader Props
title
title
Type | Default |
---|---|
String (Required) | No Default |
styles
styles
Type | Default |
---|---|
StyleSheet of type LLChatHeaderStyles | No Default, if present styles props would be applied on top of internal styles of type LLChatHeaderStyles |
styles Props
Updated 12 months ago
What’s Next