Sponsorship
A sponsor is an object that is created on the Application level in the Producer Site. Sponsor maintains a many to many relationship with Programs and Chatrooms which can be managed in the Sponsors section of your application in the Producer Site
Creating a Sponsor
- Head to your Application on the Producer Site
- Select "Sponsors" in the Sidebar
- Select the "New Sponsor" button on the top right hand side
- Enter Sponsor Name, pick a logo image to upload and select the brand color
- Select "Create" to finish
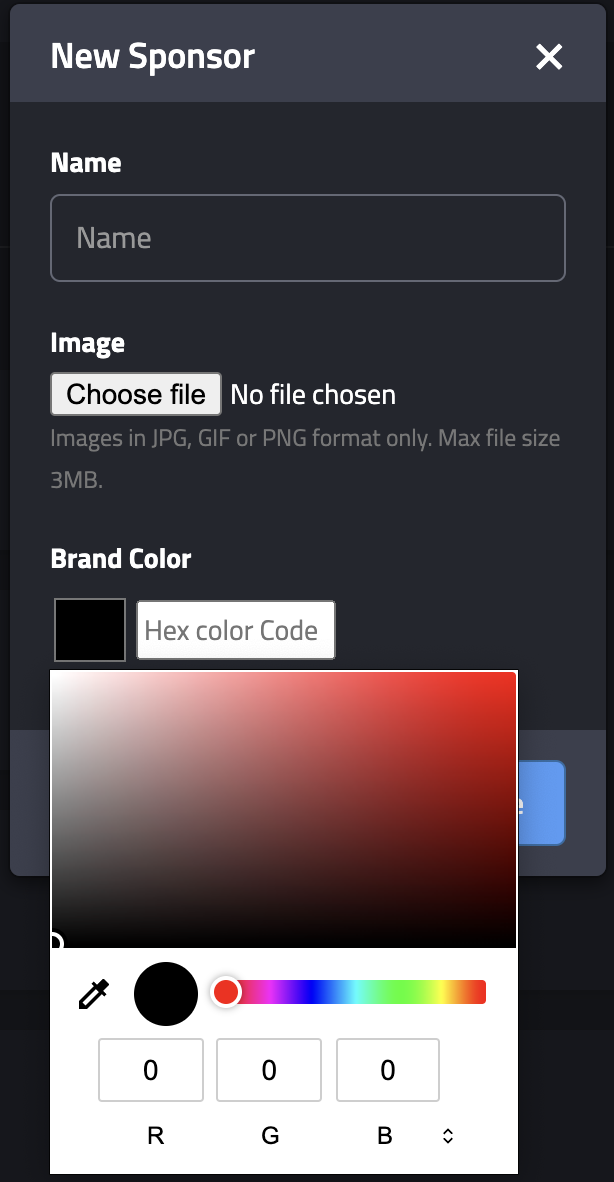
At this point you have a sponsor that is ready to be linked to a program.
Linking Sponsor(s) with a Program
- Head to your Application on the Producer Site
- Select "Programs" in the Sidebar
- Select the program you are working with
- Press on the vertical triple dots button of a program
- Select "Edit Program"
- Scroll to the "Linked Sponsors"
- Select/Unselect the Sponsor you would like to link (use ctrl select for multiple sponsor selections)
- Press "Update" to update Sponsor link changes that have been made to the program
Now you have Sponsors that are linked to a program.
Retrieving Application Sponsor(s) in the SDK
Get list of all sponsors created in a given application
LiveLike.getApplicationSponsors()
.then((paginatedSponsors) => {
console.log(paginatedSponsors);
})
sdk.sponsorship.getByApplication(page: .first) { result in
switch result {
case .success(let sponsors):
//Success Block
case .failure(let error):
//Failure Block
}
}
sdk.sponsor().fetchForApplication(LiveLikePagination.FIRST, object : LiveLikeCallback<List<SponsorModel>>() {
override fun onResponse(result: List<SponsorModel>?, error: String?) {
}
})
Retrieving Program Sponsor(s) in the SDK
Now that you have linked sponsor(s) to a program, you will now be able to retrieve sponsor information through our SDK interfaces.
LiveLike.getProgramSponsors({ programId: "<program id>"})
.then(paginatedSponsors => {
console.log(paginatedSponsors);
})
class HelloWorld {
let sdk: EngagementSDK
sdk.sponsorship.getBy(programID: <"progam id">) { result in
switch result {
case let .success(let sponsors):
//do something with sponsors
case let .failure(error):
print(error)
}
}
}
sdk.sponsor().fetchByProgramId(
<program-id>,
LiveLikePagination.FIRST,
object : LiveLikeCallback<List<SponsorModel>>() {
override fun onResponse(result: List<SponsorModel>?, error: String?) {
}
}
)
final sponsorsList =await sdk.sponsor.fetchByProgramId(<program-id>)
Retrieving Chatroom Sponsor(s) in the SDK
LiveLike.getChatRoomSponsors({ roomId: "room-id" })
.then(paginatedSponsors => {
console.log(paginatedSponsors);
})
self.sdk.sponsorship.getBy(
chatRoomID: chatroom.roomID,
page: .first,
completion: { result in
switch result {
case .success(let sponsors):
//Success Block
case .failure(let error):
//Failure Block
}
}
)
sdk.sponsor().fetchByChatRoomId(
<chat-room-id>,
LiveLikePagination.FIRST,
object : LiveLikeCallback<List<SponsorModel>>() {
override fun onResponse(result: List<SponsorModel>?, error: String?) {
}
})
Updated 5 months ago