LLChatMessageList
component represents the chat message list. Internally we render FlatList
component from react-native
.
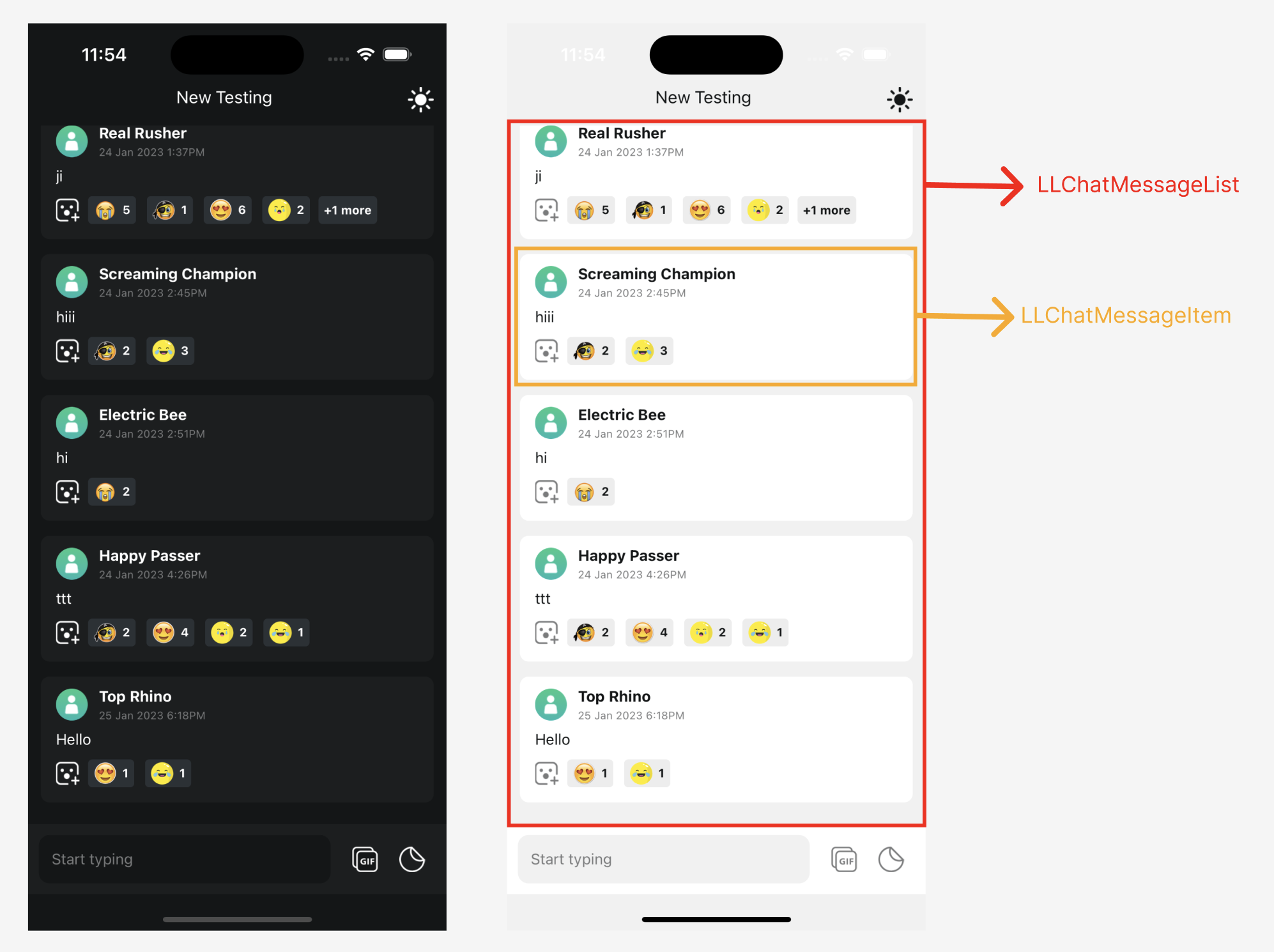
import React from 'react';
import {
LLChat,
LLChatMessageList,
LLChatMessageListStyles,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={(props) => (
<LLChatMessageList {...props} styles={messageListStyle} />
)}
/>
);
}
const messageListStyle: Partial<LLChatMessageListStyles> = StyleSheet.create({
rootContainer: { padding: 10 },
});
Type | Default |
---|
String (Required) | No Default |
import React from 'react';
import {
LLChat,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
} from '@livelike/react-native';
function MyMessageItemComponent(props: LLChatMessageItemProps) {
// render your custom chat header
}
const MyMessageListComponent = (props: LLChatMessageListProps) => {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
};
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
Type | Default |
---|
LLChatMessageItemStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItem styles. |
import React from 'react';
import {
LLChat,
LLChatMessageItemStyles,
LLChatMessageList,
LLChatMessageListProps,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
const MyMessageListComponent = (props: LLChatMessageListProps) => {
return (
<LLChatMessageList
{...props}
MessageItemComponentStyles={messageItemStyles}
/>
);
};
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
const messageItemStyles: Partial<LLChatMessageItemStyles> = StyleSheet.create({
messageItemContainer: { backgroundColor: 'darkgrey', marginVertical: 20 },
selfMessageItemContainer: { backgroundColor: 'red' },
});
import React from 'react';
import {
LLChat,
LLChatMessageList,
LLChatMessageListProps,
LLMessageListEmptyComponentProps,
} from '@livelike/react-native';
function MyMessageListEmptyComponent(props: LLMessageListEmptyComponentProps) {
// render your custom message list empty component
}
const MyMessageListComponent = (props: LLChatMessageListProps) => {
return (
<LLChatMessageList
{...props}
MessageListEmptyComponent={MyMessageListEmptyComponent}
/>
);
};
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
Type | Default |
---|
StyleSheet of type LLChatMessageListStyles | No Default, if present styles props would be applied on top of internal LLChatMessageList styles. |
CSS Class | Type | Description |
---|
rootContainer | ViewStyle | Message list container |
listLoadingIndicator | ViewStyle | Loading indicator styles |
LLChatMessageItem
represents a single message in message list and consists of header, body and footer.
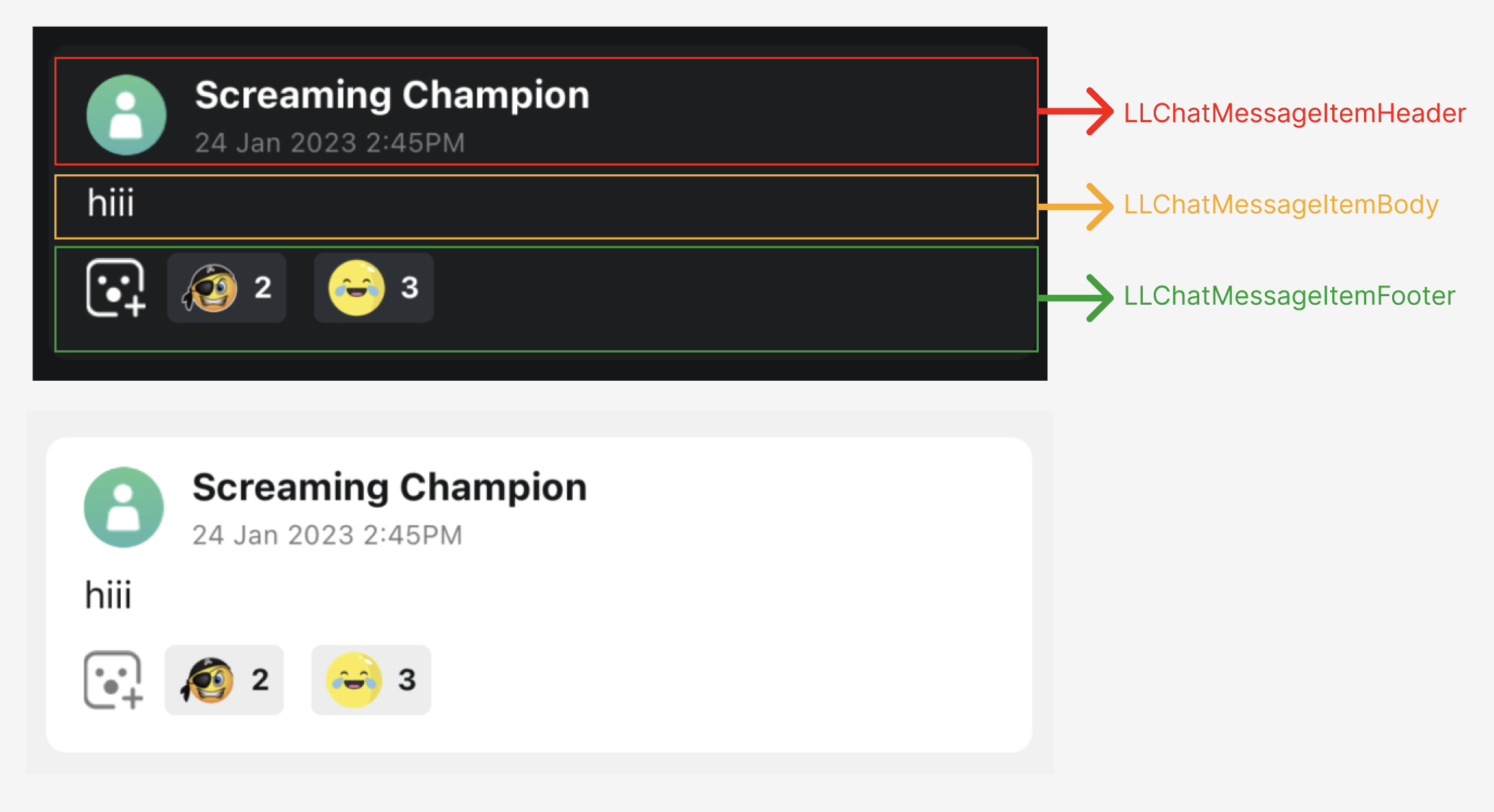
import React from 'react';
import {
LLChat,
LLChatMessageItem,
LLChatMessageItemBodyProps,
LLChatMessageItemFooterProps,
LLChatMessageItemHeaderProps,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
} from '@livelike/react-native';
function MyMessageItemComponent(props: LLChatMessageItemProps) {
return (
<LLChatMessageItem
{...props}
MessageItemHeaderComponent={(props: LLChatMessageItemHeaderProps) => {
// render your custom message item header
}}
MessageItemBodyComponent={(props: LLChatMessageItemBodyProps) => {
// render your custom message item body
}}
MessageItemFooterComponent={(props: LLChatMessageItemFooterProps) => {
// render your custom message item footer
}}
/>
);
}
const MyMessageListComponent = (props: LLChatMessageListProps) => {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
};
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
Type | Default |
---|
LLChatMessageItemBodyStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItemBody styles. |
import React from 'react';
import {
LLChat,
LLChatMessageItem,
LLChatMessageItemBodyStyles,
LLChatMessageItemFooterStyles,
LLChatMessageItemHeaderStyles,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
function MyMessageItemComponent(props: LLChatMessageItemProps) {
return (
<LLChatMessageItem
{...props}
MessageItemHeaderComponentStyles={headerStyle}
MessageItemBodyComponentStyles={bodyStyle}
MessageItemFooterComponentStyles={footerStyle}
/>
);
}
const MyMessageListComponent = (props: LLChatMessageListProps) => {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
};
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
const headerStyle: Partial<LLChatMessageItemHeaderStyles> = StyleSheet.create({
avatarImage: { height: 70, width: 70 },
username: { color: 'green' },
ownUsername: { color: 'red' },
timestamp: { marginTop: 10, fontSize: 9, color: 'green' },
ownTimestamp: { marginTop: 10, fontSize: 9, color: 'red' },
});
const bodyStyle: Partial<LLChatMessageItemBodyStyles> = StyleSheet.create({
textContainer: { margin: 10 },
});
const footerStyle: Partial<LLChatMessageItemFooterStyles> = StyleSheet.create({
footerContainer: { marginTop: 15 },
addReactionIcon: { height: 30, width: 30 },
});
LLChatMessageItemHeader
component represents the header of the message item
Type | Default |
---|
boolean (Required) | No Default |
Type | Default |
---|
(date: string) => string. | Default formatter function convertDateTime is applied. |
Type | Default |
---|
StyleSheet of type LLChatMessageItemHeaderStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItemHeader styles. |
LLChatMessageItemBody
component represents the body of the message item and renders the message text.
Type | Default |
---|
boolean (Required) | No Default |
Type | Default |
---|
StyleSheet of type LLChatMessageItemBodyTextStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItemBodyText styles. |
Type | Default |
---|
StyleSheet of type LLChatMessageItemBodyStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItemBody styles. |
CSS Class | Type | Description |
---|
textContainer | ViewStyle | Root body container |
selfMessageTextContainer | ViewStyle | Root body container for self message |
textContent | ViewStyle | Text content container |
LLChatMessageItemBody
represents the footer of the message item and renders the reaction picker and reaction count details as well
import React from 'react';
import {
LLChat,
LLChatMessageItem,
LLChatMessageItemFooter,
LLChatMessageItemFooterProps,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
LLUserReactionCountsProps,
} from '@livelike/react-native';
function MyUserReactionsCountComponent(props: LLUserReactionCountsProps) {
// render your custom user reactions component
}
function MyMessageItemFooter(props: LLChatMessageItemFooterProps) {
return (
<LLChatMessageItemFooter
{...props}
UserReactionsCountComponent={MyUserReactionsCountComponent}
/>
);
}
function MyMessageItemComponent(props: LLChatMessageItemProps) {
return (
<LLChatMessageItem
{...props}
MessageItemFooterComponent={MyMessageItemFooter}
/>
);
}
function MyMessageListComponent(props: LLChatMessageListProps) {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
}
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
Type | Default |
---|
StyleSheet of type LLUserReactionCountsStyles | No Default, if present styles props would be applied on top of internal LLUserReactionCounts styles. |
import React from 'react';
import {
LLChat,
LLChatMessageItem,
LLChatMessageItemFooter,
LLChatMessageItemFooterProps,
LLChatMessageItemProps,
LLChatMessageList,
LLChatMessageListProps,
LLUserReactionCountsStyles,
} from '@livelike/react-native';
import { StyleSheet } from 'react-native';
function MyMessageItemFooter(props: LLChatMessageItemFooterProps) {
return (
<LLChatMessageItemFooter
{...props}
UserReactionsCountComponentStyles={userReactionsCountStyles}
/>
);
}
function MyMessageItemComponent(props: LLChatMessageItemProps) {
return (
<LLChatMessageItem
{...props}
MessageItemFooterComponent={MyMessageItemFooter}
/>
);
}
function MyMessageListComponent(props: LLChatMessageListProps) {
return (
<LLChatMessageList
{...props}
MessageItemComponent={MyMessageItemComponent}
/>
);
}
export function MyApp() {
return (
<LLChat
roomId="<Your chat room id>"
MessageListComponent={MyMessageListComponent}
/>
);
}
const userReactionsCountStyles: Partial<LLUserReactionCountsStyles> =
StyleSheet.create({
reactionCountsContainer: { marginHorizontal: 10 },
moreReactionsView: { backgroundColor: 'darkgrey' },
moreReactionsText: { fontSize: 12 },
});
Type | Default |
---|
StyleSheet of type LLChatMessageItemFooterStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItemFooter styles. |
CSS Class | Type | Description |
---|
footerContainer | ViewStyle | Root footer container |
addReactionIcon | ImageStyle | Add Reaction image |
The LLChatMessageItemBodyText
component renders the message body content. It can be a text, gif or sticker.
Type | Default |
---|
boolean | No Default |
Type | Default |
---|
boolean | No Default |
Type | Default |
---|
StyleSheet of type LLChatMessageItemBodyTextStyles | No Default, if present styles props would be applied on top of internal LLChatMessageItemBodyText styles. |
CSS Class | Type | Description |
---|
text | TextStyle | Message text styles |
deletedMessageText | TextStyle | Message text for deleted message styles |
selfMessageText | TextStyle | Message text for self message styles |
stickerImage | ImageStyle | Message sticker image styles |